TypeScript is a typed superset of JavaScript that compiles to plain JavaScript. This is a list of TypeScript types for Next.js generated from the declaration files in https://github.com/zeit/next.js/tree/v10.1.3.
The one-page guide to TypeScript: usage, examples, links, snippets, and more. This is Devhints.io cheatsheets — a collection of cheatsheets I've written. React typescript reference ts cheatsheet hacktoberfest typescript-playground JavaScript MIT 1,708 23,582 2 1 Updated Apr 5, 2021 react-typescript-cheatsheet-es. Typescript Cheatsheet. My Typescript cheatsheet, which contains some frequently used basic stuff and stuff that I always forget. If you spot a mistake or want to add more cheatsheet, feel free to open an issue. Typescript Cheatsheet. Explicit and Implicit type; Common Types; Union; Array; Interface, Utility, and Object. Simple Interface; Partial; Type guard.
See also my TypeScript React cheat sheet and TypeScript cheat sheet.
- default ‹ next ‹ default ‹ createServer(function)
- GetServerSideProps<P, Q extends ParsedUrlQuery> (type)
- GetServerSidePropsContext<Q extends ParsedUrlQuery> (type)
- GetServerSidePropsResult<P> (type)
- GetStaticPaths<P extends ParsedUrlQuery> (type)
- GetStaticPathsContext(type)
- GetStaticPathsResult<P extends ParsedUrlQuery> (type)
- GetStaticProps<P, Q extends ParsedUrlQuery> (type)
- GetStaticPropsContext<Q extends ParsedUrlQuery> (type)
- GetStaticPropsResult<P> (type)
- InferGetServerSidePropsType<T> (type)
- InferGetStaticPropsType<T> (type)
- NextApiHandler ‹ NextApiHandler ‹ NextApiHandler<T> (type)
- NextApiRequest ‹ NextApiRequest ‹ NextApiRequest(interface)
- NextApiResponse ‹ NextApiResponse ‹ NextApiResponse<T> (type)
- NextComponentType ‹ NextComponentType ‹ NextComponentType<C extends BaseContext, IP, P> (type)
- NextPage<P, IP> (type)
- NextPageContext ‹ NextPageContext ‹ NextPageContext(interface)
- PageConfig(type)
- react(module)
- HtmlHTMLAttributes<T> (interface)
- LinkHTMLAttributes<T> (interface)
- StyleHTMLAttributes<T> (interface)
- Redirect(type)
- * from './dist/next-server/lib/amp'
- isInAmpMode(function)
- useAmp(function)
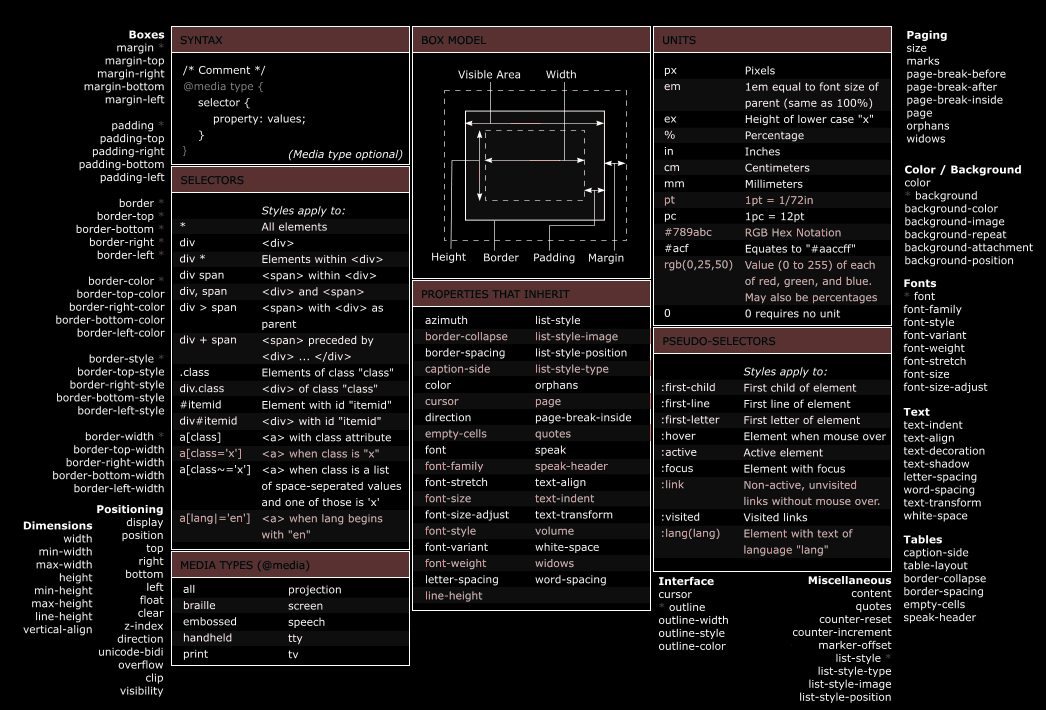
- * from './dist/pages/_app'
- AppContext(type)
- AppInitialProps ‹ AppInitialProps ‹ AppInitialProps(type)
- AppProps<P> (type)
- Container(function)
- createUrl(function)
- NextWebVitalsMetric ‹ NextWebVitalsMetric ‹ NextWebVitalsMetric(type)
- default ‹ default ‹ App(class)
- * from './dist/client/index'
- __webpack_public_path__(var)
- emitter(var)
- global(module)
- Window(interface)
- render(function)
- renderError(function)
- router(var)
- version(var)
- default ‹ default ‹ [anonymous](function)

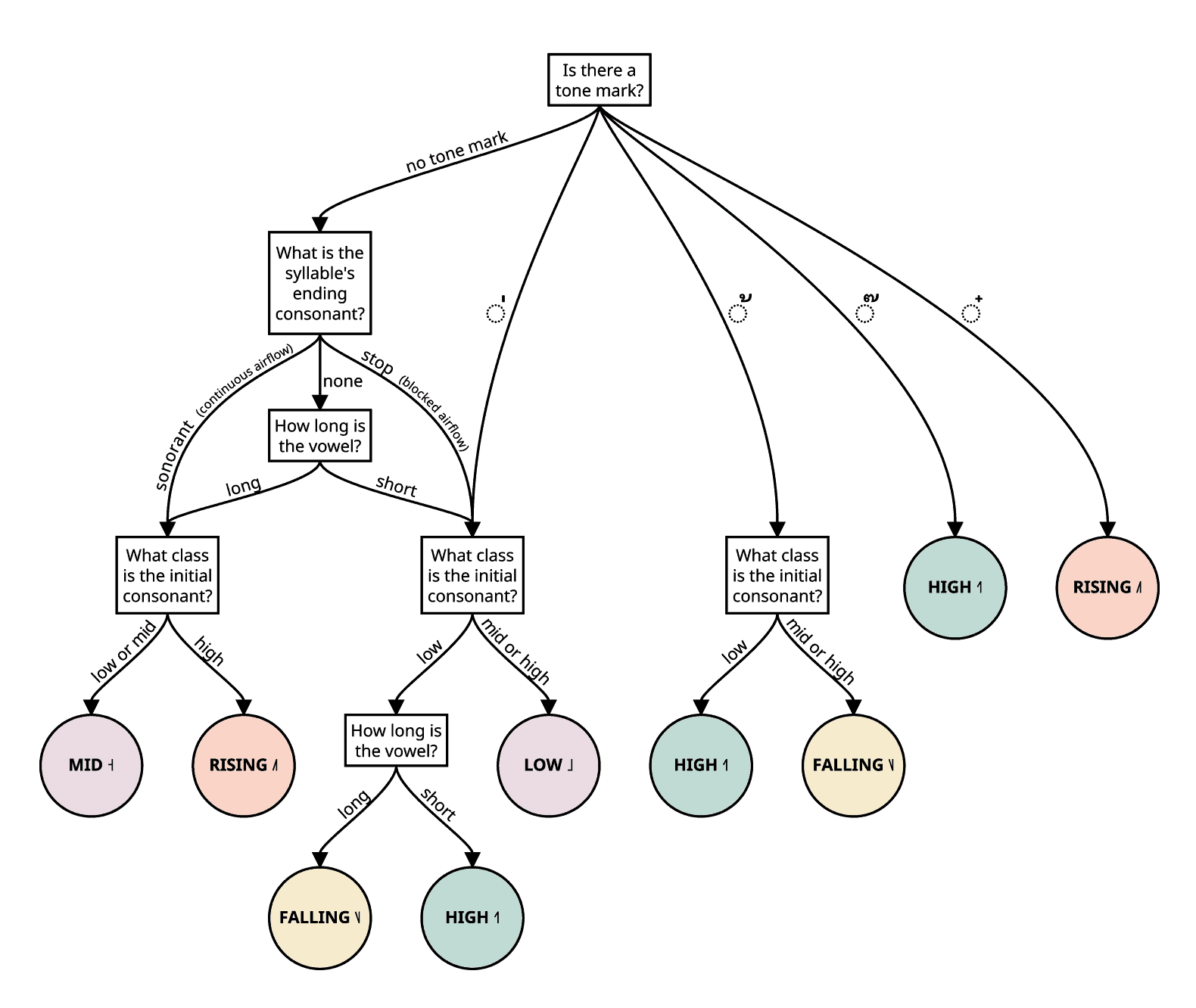
- * from './dist/next-server/lib/runtime-config'
- setConfig(function)
- default ‹ default ‹ [anonymous](function)
- * from './dist/next-server/lib/constants'
- AMP_RENDER_TARGET(var)
- BLOCKED_PAGES(var)
- BUILD_ID_FILE(var)
- BUILD_MANIFEST(var)
- CLIENT_PUBLIC_FILES_PATH(var)
- CLIENT_STATIC_FILES_PATH(var)
- CLIENT_STATIC_FILES_RUNTIME(var)
- CLIENT_STATIC_FILES_RUNTIME_AMP(var)
- CLIENT_STATIC_FILES_RUNTIME_MAIN(var)
- CLIENT_STATIC_FILES_RUNTIME_POLYFILLS(var)
- CLIENT_STATIC_FILES_RUNTIME_REACT_REFRESH(var)
- CLIENT_STATIC_FILES_RUNTIME_WEBPACK(var)
- CONFIG_FILE(var)
- DEV_CLIENT_PAGES_MANIFEST(var)
- EXPORT_DETAIL(var)
- EXPORT_MARKER(var)
- FONT_MANIFEST(var)
- IMAGES_MANIFEST(var)
- OPTIMIZED_FONT_PROVIDERS(var)
- PAGES_MANIFEST(var)
- PERMANENT_REDIRECT_STATUS(var)
- PHASE_DEVELOPMENT_SERVER(var)
- PHASE_EXPORT(var)
- PHASE_PRODUCTION_BUILD(var)
- PHASE_PRODUCTION_SERVER(var)
- PRERENDER_MANIFEST(var)
- REACT_LOADABLE_MANIFEST(var)
- ROUTES_MANIFEST(var)
- SERVER_DIRECTORY(var)
- SERVER_FILES_MANIFEST(var)
- SERVER_PROPS_ID(var)
- SERVERLESS_DIRECTORY(var)
- STATIC_PROPS_ID(var)
- STATIC_STATUS_PAGES(var)
- STRING_LITERAL_DROP_BUNDLE(var)
- TEMPORARY_REDIRECT_STATUS(var)
Typescript Cheat Sheets
- * from './dist/pages/_document'
- DocumentContext ‹ DocumentContext ‹ DocumentContext(type)
- DocumentInitialProps ‹ DocumentInitialProps ‹ DocumentInitialProps(type)
- DocumentProps ‹ DocumentProps ‹ DocumentProps(type)
- Head(class)
- Html(function)
- Main(function)
- NextScript(class)
- OriginProps(type)
- default ‹ default ‹ Document(class)
- * from './dist/next-server/lib/dynamic'
- DynamicOptions<P> (type)
- LoadableBaseOptions<P> (type)
- LoadableComponent<P> (type)
- LoadableFn<P> (type)
- LoadableGeneratedOptions(type)
- LoadableOptions<P> (type)
- Loader<P> (type)
- LoaderComponent<P> (type)
- LoaderMap(type)
- noSSR(function)
- default ‹ default ‹ dynamic(function)
- * from './dist/pages/_error'
- ErrorProps(type)
- default ‹ default ‹ Error(class)
- * from './dist/next-server/lib/head'
- defaultHead(function)
- default ‹ default ‹ Head(function)
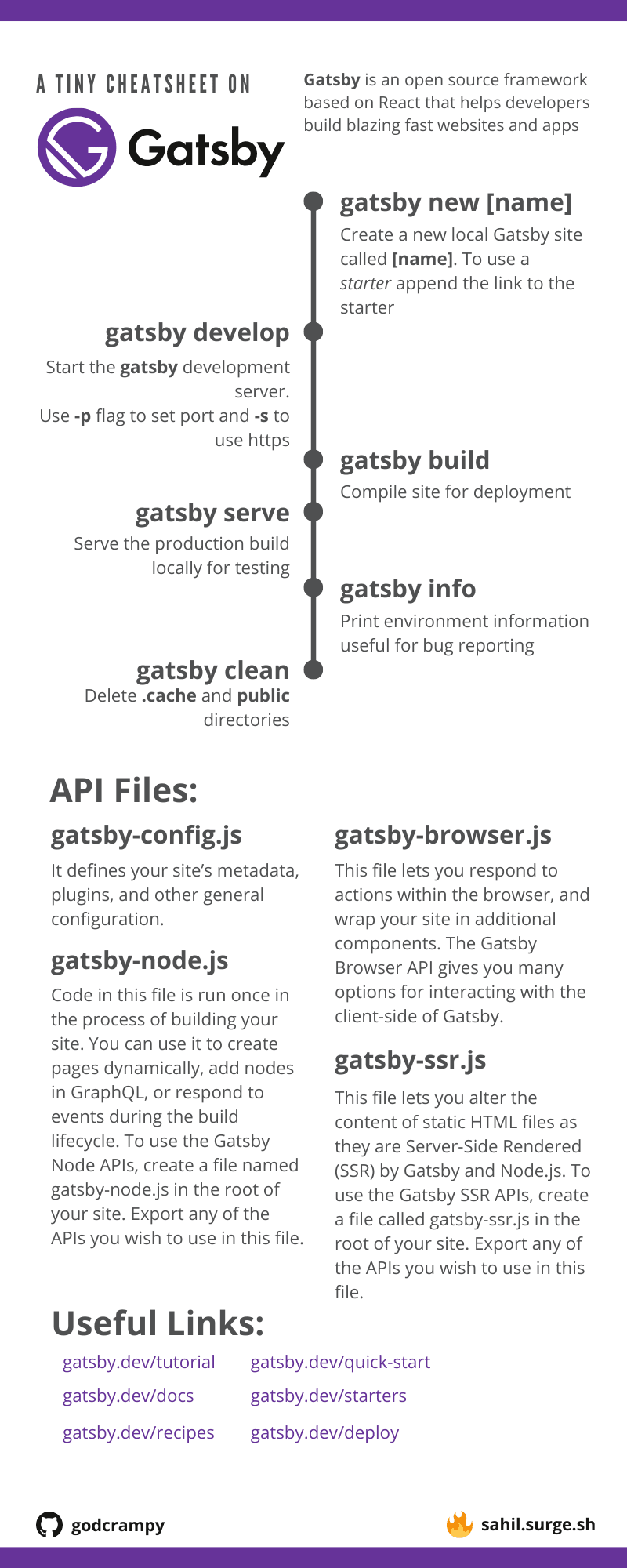
- * from './dist/client/image'
- ImageLoader(type)
- ImageLoaderProps(type)
- ImageProps(type)
- default ‹ default ‹ Image(function)
Typescript Cheat Sheet Pdf
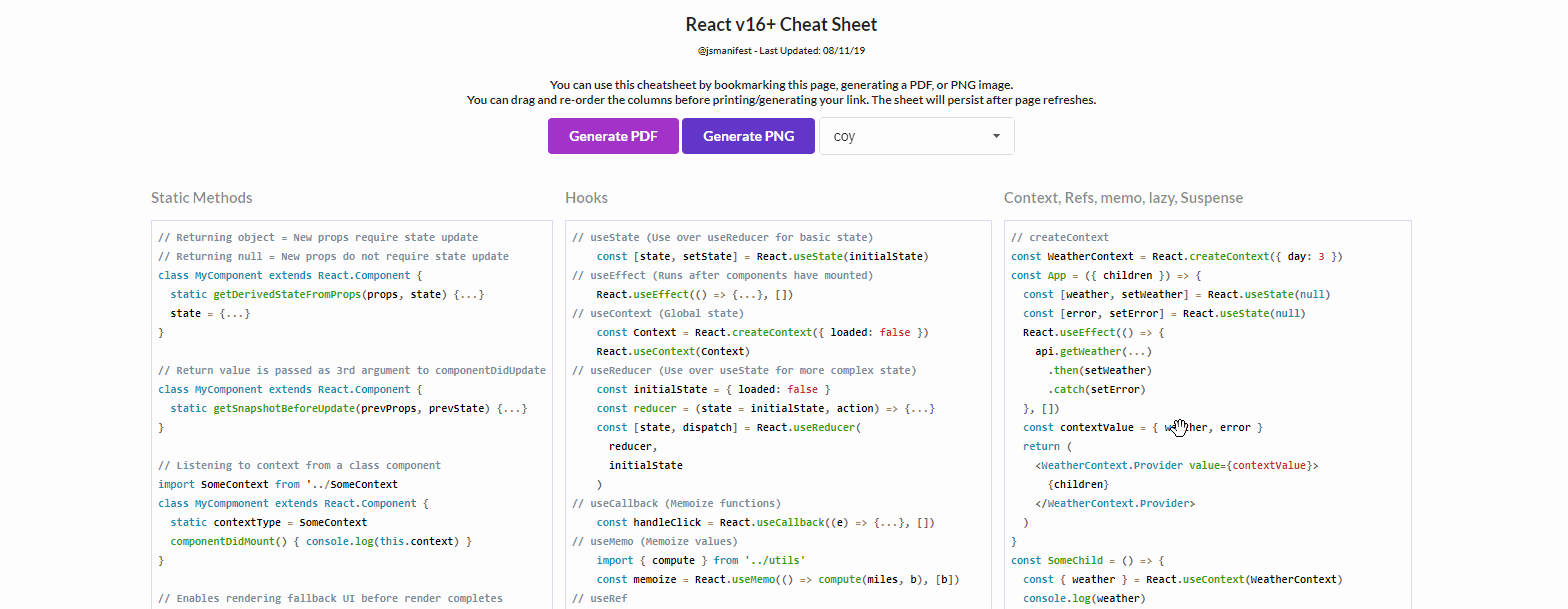
- * from './dist/client/link'
- LinkProps(type)
- default ‹ default ‹ Link(function)
Typescript Advanced
- * from './dist/client/router'
- createRouter(var)
- makePublicRouterInstance(function)
- NextRouter ‹ NextRouter ‹ NextRouter(type)
- Router ‹ Router ‹ default ‹ Router(class)
- SingletonRouter(type)
- useRouter(function)
- withRouter ‹ default ‹ withRouter(function)
- default ‹ default ‹ singletonRouter(var)
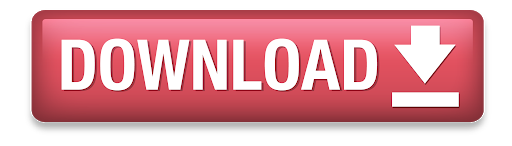